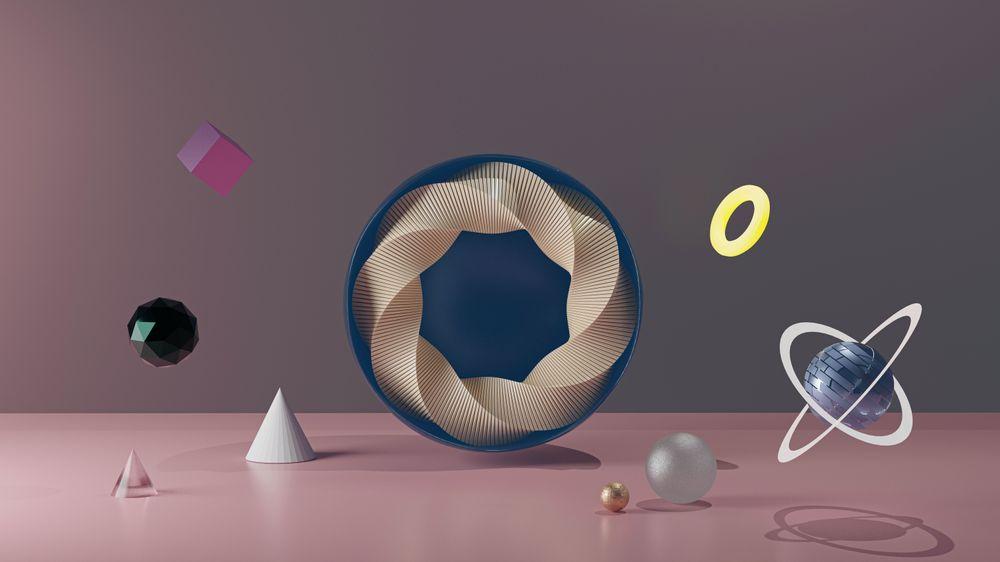
React Three Fiber for Displaying a glTF 3D Model
React Three Fiber is a React wrapper for the Three.js library, facilitating the integration of 3D objects into React applications. It claims similar speed and feature capabilities as Three.js directly. In this article, I show you by using a demo application how to display a glTF model in the browser.
glTF
GlTF is a file format for three-dimensional scenes and models. It is an open standard managed by the Khronos Group. GlTF exists as two variants, .gltf as text format and .glb as binary format. A lot of 3D software applications can directly export in glTF, for example Blender or Autodesk 3D Max.
React Three Fiber
React Three Fiber is basically a React wrapper around the Three.js library. Three.js is a popular open source library for creating and displaying 3D objects in the browser. Thus, by leveraging React Three Fiber you can more easily use 3D objects in your React application than by going directly to Three.js. It makes Three.js available in a React idiomatic way.
It claims to be as fast as using Three.js directly. Also, it offers as many features as Three.js.
Example Application
In order to demonstrate how to display a glTF 3D model using React Three Fiber we first create a React Three Fiber application using one of the ways indicated in the React Three Fiber documentation under: https://docs.pmnd.rs/react-three-fiber/getting-started/installation
Then, we download a public domain model from Smithsonian here. It is a model of the command module of Apollo 11.
You need to call a utility application called gltfjsx
which converts the .glb file into a React component, like so:
npx gltfjsx apollo_exterior-150k-4096.glb
Copy the resulting React component .jsx
file into your React application.
In your App.css
you need to adapt the available size like this:
html, body {
width: 100%;
height: 100%;
margin: 0;
}
Now modify the App.tsx
of your application to display the Apollo module:
import { Suspense } from 'react'
import './App.css'
import { Canvas } from '@react-three/fiber'
import { Model } from './Apollo';
import { OrbitControls } from '@react-three/drei';
function App() {
return (
<>
<Canvas>
<ambientLight intensity={0.5} />
<OrbitControls />
<Suspense>
<Model position={[0, -500, 0]} />
</Suspense>
</Canvas>
</>
)
}
export default App
In my case, I called the React component Apollo
and used the model component within a React Suspense
component.
Everything is displayed on a canvas and there are also some lights so that you can view the model.
The OrbitControls
component manages the rotation of the model with your mouse in the browser.
If you have not done so, you will also need to install the React library @react-three/drei
because it is also needed.
Start your application with npm run dev
and if everything went fine, you should see the Apollo 3D model. If you don't see the model at first, try rotating the model into the viewport.
It should look like this:
Use Cases
Use cases for this application would be for example e-commerce websites where you want to integrate 3D product models in your shop. If the e-commerce site is written in React, for example with Next.js, using React Three Fiber with glTF models would be a great solution. You can leverage 3D models in a React way.
Also, for example, museums that have their sites written in React and want to show some of their artifacts in 3D would benefit from using React Three Fiber.
I am sure you will come up with more use cases. Write me if you have used React Three Fiber in your project!
Conclusion
React Three Fiber offers an easy path to get started with 3D when you already have React knowledge. It leverages the power of Three.js and lets programmers integrate 3D models in their websites. This article only scratched the surface, and there is much more to explore.
References
- Kronos glTF: https://www.khronos.org/gltf
- React Three Fiber: https://docs.pmnd.rs/react-three-fiber
- gltfjsx: https://github.com/pmndrs/gltfjsx
- Command Module Apollo 11 glTF from Smothsonian (CC0): https://3d.si.edu/object/3d/command-module-apollo-11:d8c6457e-4ebc-11ea-b77f-2e728ce88125
Cover photo by Mehdi MeSSrro on Unsplash
Published
31 Aug 2023