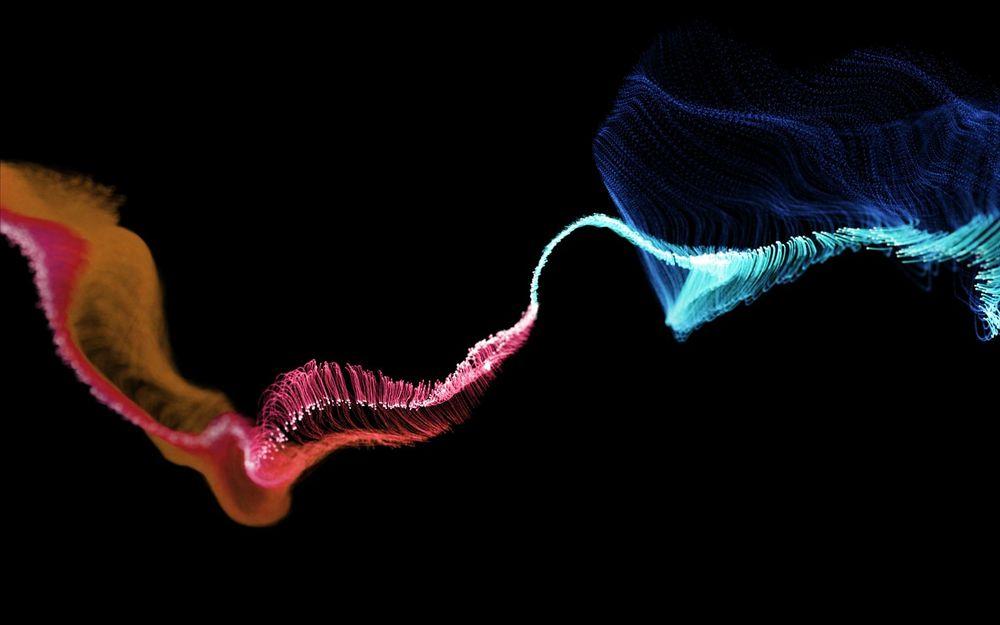
React Native Audio Recording App
React Native offers to rapidly build performant mobile apps using one JavaScript or TypeScript codebase. It is especially easy for React web developers to learn React Native. In this article, I show you how to build mobile apps that record audio and send the audio data to a Flask backend.
React Native App
For React Native developers, there exists an enhancement library with tools that make it easier to quickly build cross-platform mobile apps. It is called Expo and is the recommended way to build React Native web apps.
You can find out how to install Expo on the Expo website.
This is the Expo TypeScript app that records audio by clicking on the "Start Recording" button. When you have finished your recording, click "Stop Recording". The WAV or MP4 audio file is then sent to the Flask backend for further processing.
App.tsx
import * as React from "react";
import { Text, View, StyleSheet, Button } from "react-native";
import { Audio } from "expo-av";
import * as FileSystem from "expo-file-system";
const FLASK_BACKEND = "http://your-flask-ip:5000/audio";
export default function App() {
const [recording, setRecording] = React.useState();
const [text, setText] = React.useState("");
async function startRecording() {
try {
await Audio.requestPermissionsAsync();
await Audio.setAudioModeAsync({
allowsRecordingIOS: true,
playsInSilentModeIOS: true,
});
const { recording } = await Audio.Recording.createAsync({
android: {
extension: ".mp4",
audioEncoder: Audio.RECORDING_OPTION_ANDROID_AUDIO_ENCODER_AAC,
outputFormat: Audio.RECORDING_OPTION_ANDROID_OUTPUT_FORMAT_MPEG_4,
},
ios: {
extension: ".wav",
sampleRate: 44100,
numberOfChannels: 2,
bitRate: 128000,
audioQuality: Audio.RECORDING_OPTION_IOS_AUDIO_QUALITY_HIGH,
outputFormat: Audio.RECORDING_OPTION_IOS_OUTPUT_FORMAT_LINEARPCM,
},
});
setRecording(recording);
} catch (err) {
console.error("Failed to start recording", err);
}
}
async function stopRecording() {
setRecording(undefined);
await recording.stopAndUnloadAsync();
const uri = recording.getURI();
try {
const response = await FileSystem.uploadAsync(
FLASK_BACKEND,
uri
);
const body = JSON.parse(response.body);
setText(body.text);
} catch (err) {
console.error(err);
}
}
return (
<View style={styles.container}>
<Button
title={recording ? "Stop Recording" : "Start Recording"}
onPress={recording ? stopRecording : startRecording}
/>
<Text>{text}</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
});
Note that there are two different audio formats used for recording the audio, depending on the platform.
Flask Backend
On the backend side, this is a minimal Flask web service in Python that accepts the audio data:
app.py
from flask import Flask
from flask import request
from flask import Response
from flask_cors import CORS
from pprint import pprint
import json
app = Flask(__name__)
CORS(app)
@app.route('/audio', methods=['POST'])
def process_audio():
data = request.get_data()
data_length = request.content_length
if (data_length > 1024 * 1024 * 10):
return 'File too large!', 400
# process data here:
print ("Processing data: ", data)
return json.dumps({ "text": "Audio successfully processed!" }), 200
You could imagine doing all kinds of audio processing in this Flask application. For example, you could store the data in a cloud storage and then do some AI/ML processing on the data.
Your only limit is your imagination.
Conclusion
These two simple programs can be your template for doing amazing audio-based application on the mobile phone with support from a backend service. I hope you could gain some insights into the audio area and get you started quickly.
References
-
React Native: https://reactnative.dev/
-
Expo: https://expo.dev/
-
Flask: https://palletsprojects.com/p/flask/
Published
28 Aug 2021