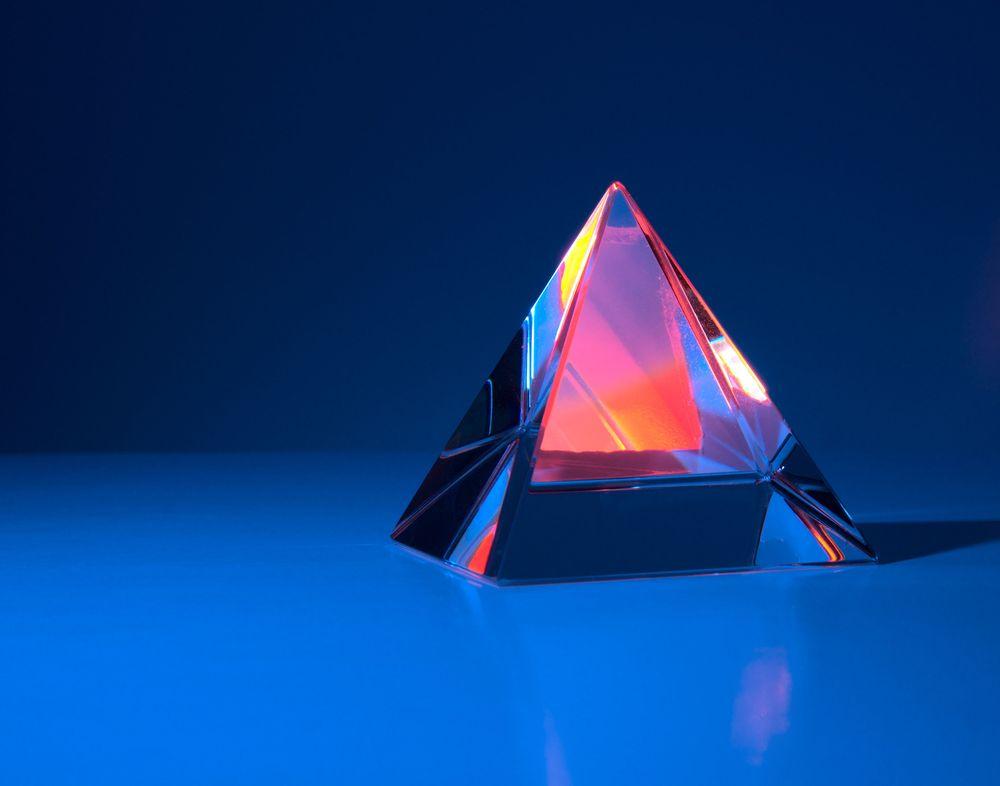
How to Dockerize a Prisma Nest.js Application
Often people use Nest.js and Prisma together when they program a backend application. Then there is the question of how to deploy the application with Docker. This article shows you how.
Nest.js
Nest.js is a framework for building server-side JavaScript and TypeScript applications. It provides an abstraction atop Express.js or Fastify. It has a good architecture and bigger server-side applications can be programmed faster than with pure Express.js or Fastify. Often, Nest.js applications use an ORM (Object-Relational Mapping) and thus Prisma comes into play.
Prisma
Using an ORM in Node and Nest.js application faciliates manipulating and querying data in a SQL database. Due to the mismatch between an object-oriented programming language like TypeScript and the relational data model, a tool like Prisma is very useful. Prisma has a single source of truth for the data model which is good design. It can easily be used within a Nest.js application.
Once you develop your Nest.js application, you also need to think about deployment. This is where containers with Docker shine.
Docker
Docker is used to automate the deployment of software in lightweight packages called containers. It is nowadays the standard of deploying server-side software in the cloud.
So when you want to deploy your Nest.js application that uses Prisma to a cloud service that supports Docker, you need to write a Dockerfile. The Dockerfile specifies the steps of creating a Docker image.
How to Dockerize
This is an example Dockerfile for a Nest.js application that uses Prisma:
# Use an official Node.js runtime as the base image
# This line specifies the base image for the Docker image. In this case, we're using an official Node.js runtime image with version 18.18 and the # Alpine Linux distribution.
FROM node:18.18-alpine
# This line sets the working directory inside the container to /usr/src/app. This is where we'll copy our application code and where the # application will run.
WORKDIR /usr/src/app
# This line installs the build-base package using the Alpine package manager (apk). This package includes the necessary tools for building
# native Node.js modules.
RUN apk add build-base
# This line installs Python 3 using the Alpine package manager. Python is required for some Node.js modules that have native dependencies.
RUN apk add python3
# This line installs the node-gyp package globally using npm. node-gyp is a tool for building native Node.js modules.
RUN npm install -g node-gyp
# Copy package.json and package-lock.json to the container
COPY package*.json ./
# Install application dependencies
RUN npm install
# Copy the rest of your application code to the container
COPY . .
# This line sets an environment variable that tells the Prisma ORM to ignore missing engine checksums. This is useful when deploying to certain
# platforms like Heroku.
ENV PRISMA_ENGINES_CHECKSUM_IGNORE_MISSING=1
# This line generates the Prisma client code based on the schema defined in the application.
RUN npx prisma generate
# Expose the port that your application will run on (Nest.js default is 3000)
EXPOSE 3000
# Define the command to run your application
CMD ["npm", "start"]
I included comments at each line of the Dockerfile. Please note that because of Prisma, there have been more steps necessary than for a pure Nest.js application. In this Dockerfile I used an Alpine Linux Node image because it is smaller in size and more secure than the standard Node image.
The next step is the build the image using Docker. You can do this with the following command:
docker build -t <image-name> .
Then you can start the image and run it as a container. If you use a tool like Docker Desktop, it is simply clicking on the run button. There also exists a command on the command line:
docker run -p <host-port>:<container-port> <image-name>
In our case, the container-port is 3000 (EXPOSE 3000 command in the Dockerfile). Finally, you should be able to access your deployed application.
Conclusion
As you could hopefully see, Nest.js applications are principally not difficult to dockerize. When using the Prisma client, there are a number of additional steps that need to be taken. I hope you learned something new.
References
-
Nest.js: https://nestjs.com
-
Prisma: https://www.prisma.io
-
Docker: https://www.docker.com
Cover photo: Michael Dziedzic on Unsplash