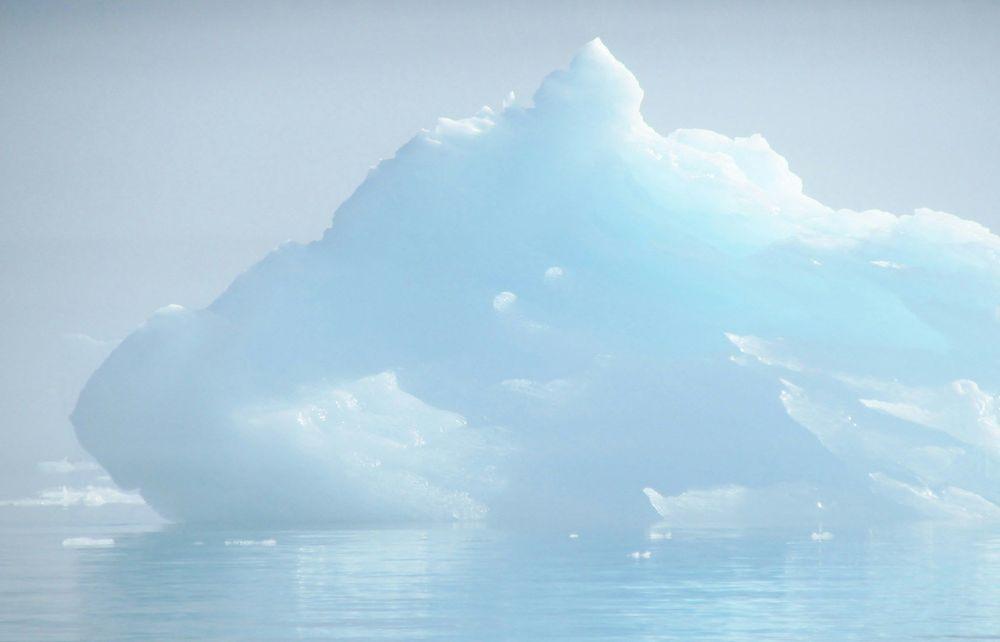
Catalog of Pure Functions in JavaScript
Pure Functions
In recent years, with the augmentation of the functional programming style in JavaScript came the insight that functions generally and especially pure functions are easier to reason about than the object-oriented programming (OOP) paradigm. Therefore, general functional style programming has become more and more prevalent in the JavaScript community. For example, React moved to function components from class components.
In computer programming, a pure function is a function that returns for identical parameters identical output. Also, a pure function has no side effects like mutation of variables or mutable arguments.
Within functional style programming, pure functions therefore take a special place. By some proponents they are the preferred way to program in JavaScript, if possible (see for example Dmitri Pavlutin).
Pure Functions in JavaScript
JavaScript as defined in the ECMAScript specification comes with a great number of predefined objects and functions. Many of the functions are not pure, but a number of them are pure functions that we can preferrably use.
These are the pure functions that are available in JavaScript by default (non-exhaustive list):
General
- parseInt(): It parses the string argument and returns an integer.
console.log(parseInt('123456'));
// 123456
- parseFloat(): It parses a string and return a floating point number.
console.log(parseFloat("3.14"));
// 3.14
String
- String.slice(): This function extracts a portion of a string and returns a new string.
const str = 'The quick brown fox jumps over the lazy dog.';
console.log(str.slice(4, 19));
// "quick brown fox"
- String.trim(): This function removes the whitespace from both ends of the string and returns a new string, not modifying the original string.
const greeting = " Hello developers! ";
console.log(greeting.trim());
// Expected output: "Hello developers!";
- String.toUpperCase(), String.toLowerCase(): Function returns the string converted to uppercase or lowercase.
const sentence = 'Hello developers!';
console.log(sentence.toUpperCase());
// Expected output: "HELLO DEVELOPERS!"
- String.substring(): This function returns the part of this string from the start index up to and excluding the end index, or to the end of the string if no end index is supplied.
const str = 'Ríoverse';
console.log(str.substring(1, 3));
// Expected output: "ío"
console.log(str.substring(2));
// Expected output: "overse"
- String.charAt(): This method returns a new string consisting of the single UTF-16 code unit at the given index.
const sentence = 'I like learning languages with Ríoverse.';
const index = 2;
console.log(`The character at index ${index} is ${sentence.charAt(index)}`);
// Expected output: "The character at index 2 is l"
- String.replace(): This method returns a new string with one, some, or all matches of a pattern replaced by a replacement.
const paragraph = "I think Tania's cat is cuter than your cat!";
console.log(paragraph.replace("Tania's", 'my'));
// Expected output: "I think my cat is cuter than your cat!"
Math
- Math.sqrt(): This method returns the square root of a number.
console.log(Math.sqrt(16));
// Expected output: 4
There are many other Math functions that are pure functions. I will leave it to a page like MDN to enumerate them all.
Array
These array functions do not modify the original array, but return a new array or a value based on the input array.
- Array.concat(): This function is used to merge two or more arrays. This method does not change the existing arrays, but instead returns a new array.
const array1 = ['α', 'β', 'γ'];
const array2 = ['d', 'e', 'f'];
const array3 = array1.concat(array2);
console.log(array3);
// Expected output: Array ["α", "β", "γ", "d", "e", "f"]
- Array.map(): This function creates a new array populated with the results of calling a provided function on every element in the calling array.
const array1 = [1, 4, 9, 16];
// Pass a function to map
const map1 = array1.map((x) => x * 2);
console.log(map1);
// Expected output: Array [2, 8, 18, 32]
- Array.filter(): The function creates a shallow copy of a portion of a given array, filtered down to just the elements from the given array that pass the test implemented by the provided function.
const words = ['spray', 'elite', 'exuberant', 'creation', 'language'];
const result = words.filter((word) => word.length > 6);
console.log(result);
// Expected output: Array ["exuberant", "creation", "language"]
- Array.reduce(): This method of the Array instances executes a user-supplied "reducer" callback function on each element of the array, in order, passing in the return value from the calculation on the preceding element. The final result of running the reducer across all elements of the array is a single value.
const array1 = [1, 2, 3, 4, 5];
// 0 + 1 + 2 + 3 + 4 + 5
const initialValue = 0;
const sumWithInitial = array1.reduce(
(accumulator, currentValue) => accumulator + currentValue,
initialValue,
);
console.log(sumWithInitial);
// Expected output: 15
- Array.every(): This method tests whether all elements in the array pass the test implemented by the provided function. It returns a Boolean value.
const isBelowThreshold = (currentValue) => currentValue < 50;
const array1 = [1, 30, 39, 29, 10, 13, 48];
console.log(array1.every(isBelowThreshold));
// Expected output: true
- Array.some(): This method tests whether at least one element in the array passes the test implemented by the provided function. It returns true if, in the array, it finds an element for which the provided function returns true; otherwise it returns false. It doesn't modify the array.
const array = [1, 2, 3, 4, 5];
// Checks whether an element is even
const even = (element) => element % 2 === 0;
console.log(array.some(even));
// Expected output: true
- Array.slice(): The method returns a shallow copy of a portion of an array into a new array object selected from start to end (end not included) where start and end represent the index of items in that array. The original array will not be modified.
const animals = ['ant', 'bison', 'camel', 'duck', 'elephant'];
console.log(animals.slice(2));
// Expected output: Array ["camel", "duck", "elephant"]
console.log(animals.slice(2, 4));
// Expected output: Array ["camel", "duck"]
Be careful, there exists a similar non-pure function called Array.splice().
- Array.toReversed(): This method the copying counterpart of the reverse() method. It returns a new array with the elements in reversed order.
const items = [1, 2, 3];
console.log(items); // [1, 2, 3]
const reversedItems = items.toReversed();
console.log(reversedItems); // [3, 2, 1]
console.log(items); // [1, 2, 3]
Note that the Array.reverse() method is the non-pure counterpart to Array.toReversed().
Object
- Object.keys(): This method returns an array of a given object's own enumerable string-keyed property names.
const object1 = {
a: 'astring',
b: 42,
c: false,
};
console.log(Object.keys(object1));
// Expected output: Array ["a", "b", "c"]
- Object.values(): The method returns an array of a given object's own enumerable string-keyed property values.
const object1 = {
a: 'somestring',
b: 42,
c: false,
};
console.log(Object.values(object1));
// Expected output: Array ["somestring", 42, false]
Conclusion
If you prefer the function style of programming in JavaScript, I am sure these pure functions will come handy in your projects. They reduce complexity and allow you to reason about your code more easily. Thus, this helps to reduce bugs.
If you have other examples of pure functions that you would like mentioned, please let me know and click the contact button below.
References
- MDN: https://developer.mozilla.org/en-US
- Blog by Dmitri Pavlutin: https://dmitripavlutin.com/javascript-pure-function
Cover Image: Photo by James Ahlberg on Unsplash
Published
25 Jan 2024