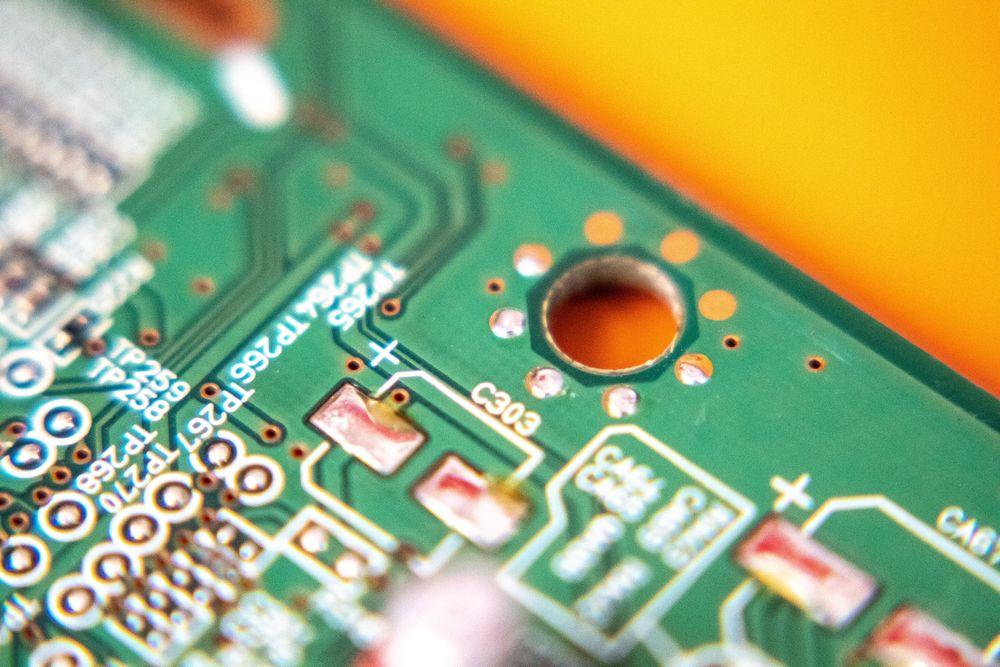
Arduino Blinking LED in Pure C
The Arduino IDE contains a simplified language for developing for the Arduino platform. This language is called Wiring and is a simplified dialect version of C and C++. Common input and output operations are simplified, making the environment ideal for prototyping for designers and makers.
But sometimes you want to program in "real" C and use the AVR tool chain directly. For example, you might want to look behind the scenes or need to program a library. Then it becomes necessary to use the AVR tool chain.
How to program a simple blinking LED in pure C, I want to show in this article.
Installation
In order to be able to compile and run the C program to AVR machine code, you need to install a cross-compiler, a linker and an uploader for the AVR microcontroller.
Under Ubuntu Linux, this done by running the following command:
sudo apt-get install gcc-avr binutils-avr gdb-avr avr-libc avrdude make
This installs all the required tool chain for AVR C development on your machine.
Arduino Hardware Setup
The C program assumes you have an Arduino Uno with one LED connected to port 13.
This Fritzing image shows the setup:
Led.c
#include <avr/io.h>
#define F_CPU 16000000
#define BLINK_DELAY_MS 5000
#include <util/delay.h>
int main (void)
{
// Arduino digital pin 13 (pin 5 of PORTB) for output
DDRB |= 0B100000; // PORTB5
while(1) {
// turn LED on
PORTB |= 0B100000; // PORTB5
_delay_ms(BLINK_DELAY_MS);
// turn LED off
PORTB &= ~ 0B100000; // PORTB5
_delay_ms(BLINK_DELAY_MS);
}
}
In this C example, the LED is turned on for 5000 ms (5 sec) and is then turned off again. You need to import two header files, one for the IO ports definition and the other for the delay function.
It is important that under pure C you need to calculate the exact pin where you want to write to. In our case, the LED pin 13 is on Port B5 (or in binary: 0B100000). You can find out about the raw port and pins in the Arduino schematics that can be accessed from the Arduino website.
Compiling and Uploading
With the attached Makefile, it becomes easy to compile the C file.
Just run:
make
Behind the scenes, the Makefile calls the AVR C compiler (avr-gcc) and converts the file to the correct hex format that the uploader can use. Before you can upload, you need to find out where on your machine the Arduino Uno is connected to. The path is then written in the Makefile as variable ARDUINO_USB.
Once that is done, upload the file to your Arduino Uno with:
make deploy
The uploader we use in the Makefile is called Avrdude.
Conclusion
The Arduino family is a great hardware prototyping playing field. But you can dive deeper and program the Arduino Uno without the Arduino IDE directly by using AVR C. I hope I could take away the first hurdles in this adventure.
References
- Led in pure C project source: https://github.com/tderflinger/arduino-blink-purec
- Arduino Uno schematics: https://www.arduino.cc/en/uploads/Main/arduino-uno-schematic.pdf
- AVR Toolchain: http://nongnu.org/avr-libc/
- Thanks to Sagar: https://github.com/sagarsp/arduino-blink-c
For best quality, watch it via this link here: https://www.youtube.com/watch?v=IP4Ikw3KtAU
Cover image by Brian Wangenheim on Unsplash
Published
5 Feb 2020